Full Stack Website Guide
published: (updated: )
by Harshvardhan J. Pandit
is part of: web development
back-end front-end server web-dev website
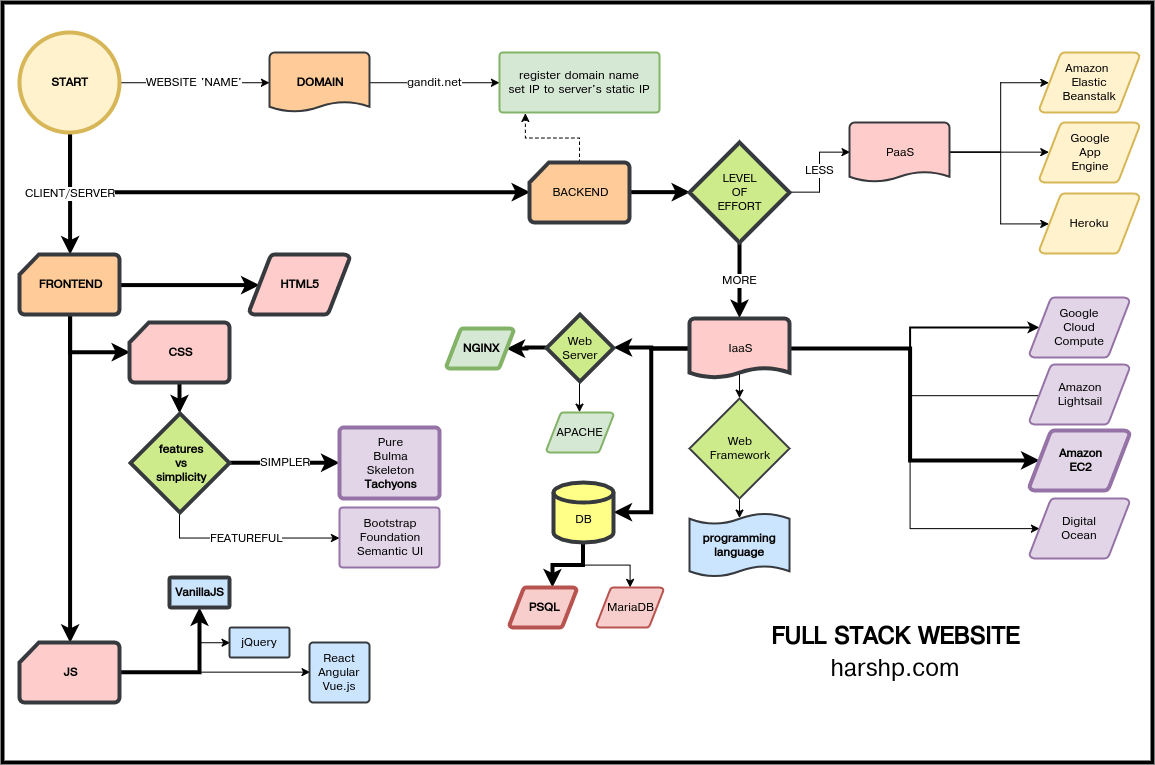
There is often the case about starting something where one wishes that they had received some kind of advice or information about the topic. Kind of an overview of what was what, and how everything fit together. The 'big picture'. This post is something similar on building a website. Over time, I have realised that there are a lot of components that are not necessarily related, but are part of the big picture. Without following any particular tutorial, I just wanted to get an idea of what was what before actually starting anything. This post hopes to serve that itch by providing some brief explanations of how things stand. Hopefully, this is helpful.
Full-stack means two components - server (backend) and client (frontend). The backend server is responsible for handling requests, which means deciding how the URL typed into the bar is handled and the correct page is sent back to the browser. The frontend is responsible for taking the content and displaying it in the browser. These are oversimplifications as the backend and frontend do a lot of other work as well.
Domain
The domain is the website, to put it concisely. It is the part of the website that the browser goes looking for, and once it has found it, asks it for specific pages. TO get a domain name, you can turn to any registrar, though I am partial to gandi.net as I have an account with them and have found them to be competitively priced with excellent support. With a domain name, you can set up subdomains such that alpha.website.com is handled by one server while beta.website.com is handled by another.
HTTPS/SSL
This is the part where the domain name begins with https://
instead of http
.
The extra 's' at the end signifies secure, and informs that traffic to and from the
website is encrypted. In this day and age, there is NO reason to NOT use HTTPS.
LetsEncrypt offers FREE SSL certificates, and they
are a breeze to set up and renew. USE THEM. You may want to investigate this area
a little more if you are doing payments, shopping, or anything that requires extra
security on your website. But if it is just a simple website, then definitely
go and get that HTTPS in there. Again, it's free, so the only excuse you have
is that you are lazy.
Backend
Choosing the infrastructure
There are two options depending on how much you want out of it and how much work you are willing to put. If you don't need the hassle of managing a server, you can choose PaaS (Platform as a Service) which means that someone else handles all the work of running a service for you. Or if you are comfortable handling your own server (which is a linux machine) then you opt for IaaS (Infrastructure as a Service) where the company provides all the components and you combine them as you want. Think of it like PaaS being like a pre-made car that you can use as long as you know driving and IaaS being like given the engine, chassis, wheels, and other components where you have to engineer a car to your needs and then use it.
PaaS
The top two PaaS offerings are Elastic Beanstalk and Google App Engine. Of these, Google App Engine is much more documented and has better pricing options. The nice thing about PaaS is that you only have to focus on how your pages are being generated (more on that later), whereas with IaaS you have to worry about things like how the database is stored and configured, how much RAM you are using, how the web server is being utilised - so a lot of low level details. There is also heroku which offers free instances with a caveat on how much time they can be used for (in a day).
IaaS
In IaaS, you get a virtual machine (server) which is mostly a linux box configured up to act as a processor with some specified RAM. I've been running my website (this one, yes) for more than a year now on AWS or Amazon Web Services or more specifically EC2. Recently, I had a go at porting it to Google Cloud or Google Compute Engine. With these, I can select a pre-configured VM (1 vCPU) and RAM (0.5GB) and the provider will provision these for me. Apart from these, there are also DigitalOcean and Amazon Lightsail which offer IaaS in pre-configured setups which cannot be changed (or mixed and matched).
Setting up the domain
When these instances are provisioned or provided, they are given an internal IP address which makes them accessible from within the provider's network. This internal IP can change which makes it non-ideal for web stuff because the website needs a fixed address. To get these working with our domain, we need a static IP address, which means that it won't change. In some providers, this can cost extra, but most of them provide one free of charge with a IaaS instance.
Web Server
Once you have an IaaS instance, you need to configure how the page requests are handled, what are valid URLs and so on. For this a web server such as Apache or Nginx (pronounced engineX) come in handy. These sit in as the first recipient of requests that come in to the server. Traditionally, Apache rules the roost of web servers. However, I use Nginx as it is easy to configure and set up.
You can have multiple 'apps' for different URLs. For example, you can configure website.com/blog to be handled by a different app than website.com/project. The interaction between the web server and a web framework happens through a port or a socket. Generally, it is preferably to have sockets as they can be configured to have better security in terms of access.
Web Framework
A web framework is a collection of utilities (or software, take your pick of a fancy term) which handles the page request after the web server has decided that the URL request is valid. This is where your favourite programming language comes in. There exist web frameworks for pretty much all languages, even C. I use Django, which is a python web framework. I also use other python-based frameworks such as flask and bottle.
The web framework is the place where you get control of the actual request and how to handle it. Things such as storing and retrieving requests from a database, or whether to return a 404 or text content. Some web frameworks are heavy or featureful in a way similar to how IaaS and PaaS compare. Some are bare bones and require you to add/mix components. Or there is a third simpler option of using a static HTML file generator that will generate all the files once (so no random component) and then you serve it using any method from IaaS or PaaS. One good static HTML generator is Jekyll.
Start with the programming language. What's your favourite programming language? Is it java? Or perhaps ruby? Or python? Or c#? Whichever it is, that will decide what web framework you are using. If you're in this for a learning experience, go for a minimal framework. Read around. See what people say. Most often, people will comment (appreciatively) about how they find a minimal framework better because they can add just the features they want. For e.g. Django comes with database ORM built in, which makes database handling a breeze. Flask, by comparison, does not come with any ORM. But if I wanted to, I could add one using SQLAlchemy. If you want to get up and running as quickly as possible, look for what 'beginners' should use - that's usually the most easy and quick to set up web framework.
Database
The craze for NoSQL
You don't need NoSQL. Let me repeat that again. YOU DON'T NEED NoSQL. Unless you really do, of course. It is more of a hyped term that has caught on and people want to be on the gravy train. The thing with NoSQL is that it is good for really niche cases which 99% websites don't need. So stick with a general RDBMS, and they're well oiled with web frameworks and web servers and services, so you can work with them out of the box.
Okay, which database?
PostgreSQL, of course. It is open, free, and fully compliant. If for some reason, you feel the need for MySQL, please instead opt to use MariaDB, an open fork that is better IMHO.
Frontend
Now comes the part where the website is displayed in a browser. The frontend is essentially how you decide the website is displayed. My philosophy is always to use something only when it is really needed. So my first opinion would be to not decide on using react or angular just because everyone seems to be using it.
Think of the basics. HTML + CSS + JS
. HTML by itself means
HTML5 now.
Beyond that, you don't need anything. The others, however, need better
considerations.
CSS Frameworks
There's a repeating pattern here, of things that work great 'out-of-the-box', but are 'big' and 'clunky'. In CSS, you have heavyweight frameworks such as Bootstrap, Foundation, and Semantic UI which have enjoyed years of popularity and use. Apart from these, there are lightweight frameworks such as Bulma, Pure, Skeleton, Tachyons, and so many others that work well at a minimalist level. Personally, I use Tachyons, which is a fancy way to write inline CSS. It works for my preferences, which is to enable writing CSS when I write blog posts. There are other better posts describing how to select CSS frameworks, so I shall not presume about adding any wisdom to them.
JS Frameworks
The hot area of current trendy conversations. It used to be JQuery just a few years back. Now there are so many options that it becomes confusing just how to differentiate their aims and applications from each other.
There is a good website, you might not need jquery that explains in quite an unusual way how JavaScript by itself is a powerful language and can do what you want concisely, or other smaller libraries that offer just the right feature-set so that you don't have to use jquery, which is 'heavy'. BTW, using plain javascript is now called VanillaJS because pure javascript just had to jump on the fancy naming bandwaggon (that's a gag/parody/satire).
Frameworks such as React and Angular solve a problem, so if you don't have that problem, don't create another one by using them. That being said, they are great to use. Another interesting framework is Vue.js which is lighter than React and Angular, and offers similar features.
If all you want is a decent looking website / blog, then plain javascript and a simpler CSS framework should suffice. At most, you can go for jquery (or the other minimal libraries that do the work you need). If you have complex needs such as dynamic views, and shopping carts, and analytics, and dashboards; then it is a good idea to familiarise yourself with what the other frameworks do before taking the plunge.
Footnote: This is not meant to be an exhaustive guide or tutorial. I wrote this down more as a documentation of what goes around whenever someone starts this discussion about full stack tools.